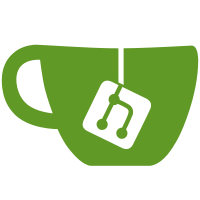
To guarantee that the 5mS pulse from a BLTouch is recognized you need to have the endstops.update() routine run twice in that 5mS period. At 200 steps per mm, my system has problems below a feedrate of 120 mm per minute. Two things were done to guarantee the two updates within 5mS: 1) In interrupt mode, a check was added to the temperature ISR. If the endstop interrupt flag/counter is active then it'll kick off the endstop update routine every 1mS until the flag/counter is zero. This flag/counter is decremented by the temperature ISR AND by the stepper ISR. 2) In poling mode, code was added to the stepper ISR that will make sure the ISR runs about every 1.5mS. The "extra" ISR runs only check the endstops. This was done by grabbing the intended ISR delay and, if it's over 2.0mS, splitting the intended delay into multiple smaller delays. The first delay can be up to 2.0mS, the next ones 1.5mS (as needed) and the last no less than 0.5mS. ========================================= BLTouch error state recovery If BLTouch already active when deploying the probe then try to reset it & clear the probe. If that doesn't fix it then declare an error. Also added BLTouch init routine to startup section
103 lines
2.8 KiB
C++
103 lines
2.8 KiB
C++
/**
|
|
* Marlin 3D Printer Firmware
|
|
* Copyright (C) 2016 MarlinFirmware [https://github.com/MarlinFirmware/Marlin]
|
|
*
|
|
* Based on Sprinter and grbl.
|
|
* Copyright (C) 2011 Camiel Gubbels / Erik van der Zalm
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*/
|
|
|
|
/**
|
|
* endstops.h - manages endstops
|
|
*/
|
|
|
|
#ifndef ENDSTOPS_H
|
|
#define ENDSTOPS_H
|
|
|
|
#include "enum.h"
|
|
|
|
class Endstops {
|
|
|
|
public:
|
|
|
|
static bool enabled, enabled_globally;
|
|
static volatile char endstop_hit_bits; // use X_MIN, Y_MIN, Z_MIN and Z_MIN_PROBE as BIT value
|
|
|
|
#if ENABLED(Z_DUAL_ENDSTOPS)
|
|
static uint16_t
|
|
#else
|
|
static byte
|
|
#endif
|
|
current_endstop_bits, old_endstop_bits;
|
|
|
|
Endstops() {};
|
|
|
|
/**
|
|
* Initialize the endstop pins
|
|
*/
|
|
void init();
|
|
|
|
/**
|
|
* Update the endstops bits from the pins
|
|
*/
|
|
static void update();
|
|
|
|
/**
|
|
* Print an error message reporting the position when the endstops were last hit.
|
|
*/
|
|
static void report_state(); //call from somewhere to create an serial error message with the locations the endstops where hit, in case they were triggered
|
|
|
|
/**
|
|
* Report endstop positions in response to M119
|
|
*/
|
|
static void M119();
|
|
|
|
// Enable / disable endstop checking globally
|
|
static void enable_globally(bool onoff=true) { enabled_globally = enabled = onoff; }
|
|
|
|
// Enable / disable endstop checking
|
|
static void enable(bool onoff=true) { enabled = onoff; }
|
|
|
|
// Disable / Enable endstops based on ENSTOPS_ONLY_FOR_HOMING and global enable
|
|
static void not_homing() { enabled = enabled_globally; }
|
|
|
|
// Clear endstops (i.e., they were hit intentionally) to suppress the report
|
|
static void hit_on_purpose() { endstop_hit_bits = 0; }
|
|
|
|
// Enable / disable endstop z-probe checking
|
|
#if HAS_BED_PROBE
|
|
static volatile bool z_probe_enabled;
|
|
static void enable_z_probe(bool onoff=true) { z_probe_enabled = onoff; }
|
|
#endif
|
|
|
|
private:
|
|
|
|
#if ENABLED(Z_DUAL_ENDSTOPS)
|
|
static void test_dual_z_endstops(const EndstopEnum es1, const EndstopEnum es2);
|
|
#endif
|
|
};
|
|
|
|
extern Endstops endstops;
|
|
|
|
#if HAS_BED_PROBE
|
|
#define ENDSTOPS_ENABLED (endstops.enabled || endstops.z_probe_enabled)
|
|
#else
|
|
#define ENDSTOPS_ENABLED endstops.enabled
|
|
#endif
|
|
|
|
|
|
#endif // ENDSTOPS_H
|